UBM Push Event API
In some instances, certain elements may not be recorded during mining due to their customized nature. To address this issue, users can utilize the Push API within UBM. This API allows users to customize areas themselves and record mining for those elements.
However, if UBM and recording are disabled within the application, events should not be recorded or pushed via custom code Push API.
The events will be sent based on the user-defined limit specified in the "Events to Cache" field of the UBM Configuration in the portal.
API Code:
window.appnaviApi.analytics.pushEvent({type: "ubm", events: [ubmEvent]});
Configuration:
let ubmEvent = {
eventType: "RIGHT_CLICK",
areaLabel: "This is area",
elementLabel: "This is element label",
tagType: window.appnaviApi.tagType.button
}
ubmEvent is an array and can be sent multiple events at a time.
let ubmEvent1 = {
eventType: "RIGHT_CLICK",
areaLabel: "area one",
elementLabel: "element one",
tagType: window.appnaviApi.tagType.button
}
let ubmEvent2 = {
eventType: "LEFT_CLICK",
areaLabel: "area two",
elementLabel: "element two",
tagType: window.appnaviApi.tagType.div
}
window.appnaviApi.analytics.pushEvent({type: "ubm",events: [ubmEvent1, ubmEvent2]
})
PROPERTIES
Following properties will be require to add in custom code to sent UBM record via Push API.
- Area Label
- Element Label
- Event type
- Tag type
UBM EVENTS
Only these events will consider to be sent via push API.
- RIGHT_CLICK
- LEFT_CLICK
TAG TYPE
Users should include the tag type in the custom code as shown below:
tagType: window.appnaviApi.tagType.button
Various tag types are available, and users can select the appropriate one based on their element.
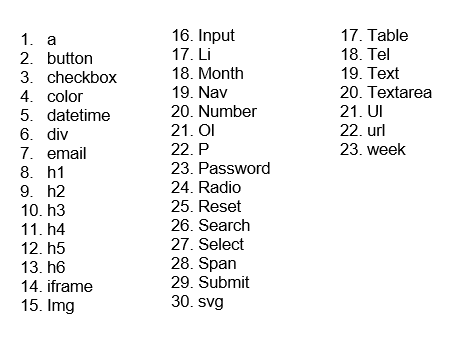
Example of setting custom Code for Push API
Suppose user wants to set custom code for first language field and for login button in following example.
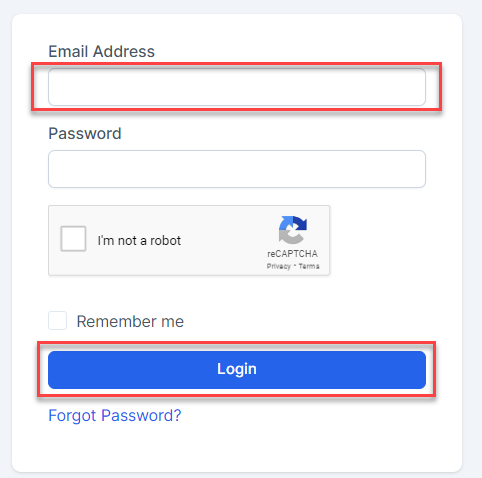
User then go to manage application and add following custom code on the custom area of application and save it.
console.log("Custom Code executed")
Array.from(document.querySelectorAll("button[title='English']")).forEach((x) => {
console.log(x, "Element")
x.addEventListener('mouseup', function(event) {
let eventType = "LEFT_CLICK"
// Check if it's a left click
if (event.which === 1 || event.button === 0) {
eventType = "LEFT_CLICK"
}
// Check if it's a right click
else if (event.which === 3 || event.button === 2) {
eventType = "RIGHT_CLICK"
}
let ubmEvent = {
eventType,
areaLabel: "my code area",
elementLabel: "my element 1",
tagType: window.appnaviApi.tagType.button
}
window.appnaviApi.analytics.pushEvent({type: "ubm",events: [ubmEvent]})
});
})
Array.from(document.querySelectorAll("button[type='submit']")).forEach((x) => {
console.log(x, "Element")
x.addEventListener('mouseup', function(event) {
let eventType = "MOUSE_OVER"
// Check if it's a left click
if (event.which === 1 || event.button === 0) {
eventType = "MOUSE_OVER"
}
// Check if it's a right click
else if (event.which === 3 || event.button === 2) {
eventType = "MOUSE_OVER"
}
let ubmEvent = {
eventType,
areaLabel: "my code area 2",
elementLabel: "my element 2 BUTTON",
tagType: window.appnaviApi.tagType.button
}
window.appnaviApi.analytics.pushEvent({type: "ubm",events: [ubmEvent]})
});
})
After adding the code, navigate to Analytics -> UBM and enable User Behavior Mining and Recording for the respective application. Additionally, adjust the "Events to Cache" setting if necessary. Save the changes and proceed to the client side.
In the provided example, if the user clicks on the language field, the event will be recorded successfully. However, if the user moves to the login button and clicks on it, a validation error will be displayed on the console indicating that "MOUSE_OVER" is an invalid event type, and consequently, the event will not be recorded.
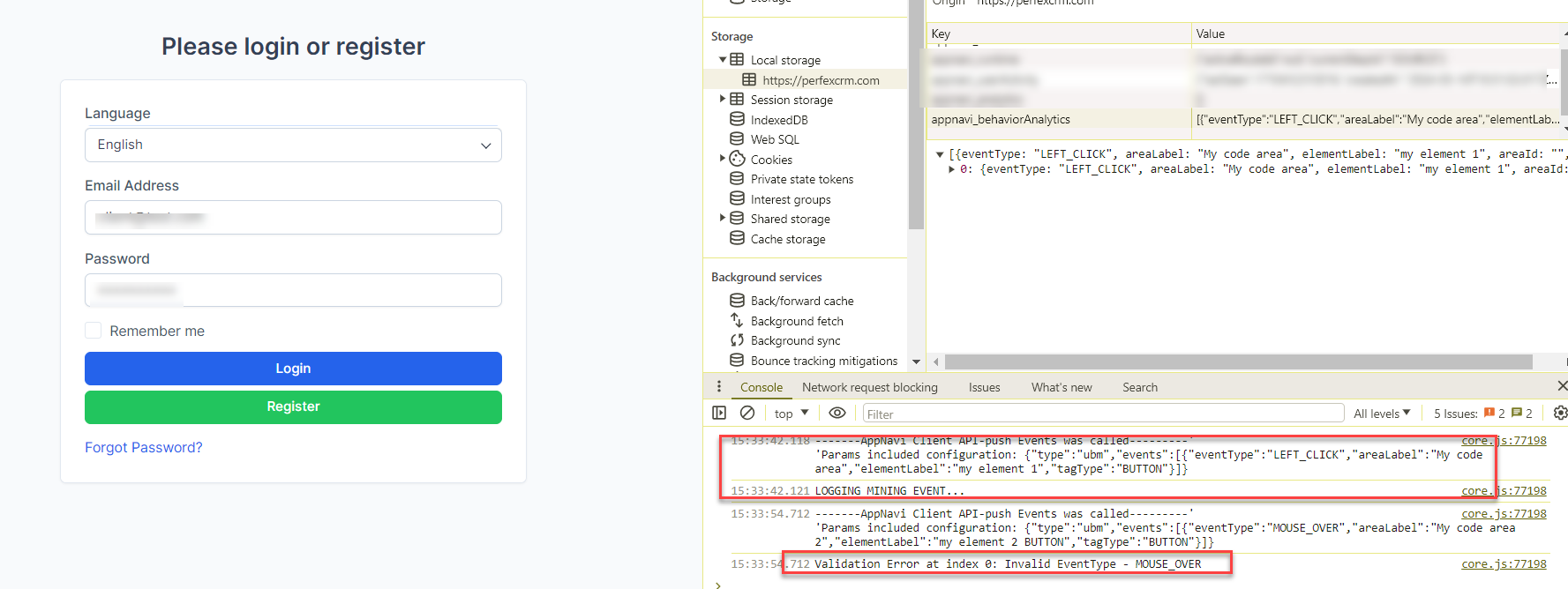
Updated about 1 month ago